modal - popup
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="https://ajax.aspnetcdn.com/ajax/jQuery/jquery-3.6.0.min.js"></script>
<style>
#sbox{
width: 200px;
height: 60px;
background-color: tomato;
cursor: pointer;
}
#popup{
width: 300px;
height: 200px;
background-color: #fff;
border: 3px solid #ddd;
border-radius: 10px;
top: 50%;
left: 50%;
position: absolute;
margin-left: -150px;
margin-top: -100px;
display: flex;
flex-flow: column nowrap;
text-align: center;
}
#popup>button{
background-color: #ccc;
padding: 5px 0;
margin: 0 20px;
border: 0;
}
</style>
<script>
$(document).ready(function(){
$("#popup").hide();
$('#sbox').click(function(){
$('#popup').fadeIn();
});
$("button").click(function(){
$("#popup").fadeOut();
});
});
</script>
</head>
<body>
<div id="sbox"><h6>누르면 popup</h6></div>
<div id="popup">
<h2>공지</h2>
<p>사이트 서버 점검으로<br>10:00~18:00까지<br>서비스 중지됩니다.</p>
<button>닫기</button>
</div>
</body>
</html>
notice-tab
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="https://ajax.aspnetcdn.com/ajax/jQuery/jquery-3.6.0.min.js"></script>
<style>
*{
margin: 0;
padding: 0;
font-family: "맑은 고딕";
font-size: 12px;
color: #666;
}
ul, li, ol{
list-style: none;
}
a{
text-decoration: none;
}
#notice{
width: 300px;
height: 190px;
margin: 20px;
}
#notice ul{
height: 32px;
background-color: #eee;
width: 240px;
}
#notice ul li{
float: left;
padding-top: 7px;
width: 80px;
height: 25px;
text-align: center;
cursor: pointer;
}
.tab_list{
float: left;
width: 280px;
height: 150px;
border-top: 2px solid #efb300;
border-bottom: 1px solid #ddd;
border-right: 1px solid #ddd;
border-left: 1px solid #ddd;
padding: 10px;
}
.port_back{
color: #fff;
background-color: #efb300;
}
.tab_sub1{
display: block;
}
.tab_sub2, .tab_sub3{
display: none;
}
</style>
<script>
$(document).ready(function(){
$("#tab_menu1").click(function(){
$(".tab_sub1").show();
$(".tab_sub2, .tab_sub3").hide();
$("#tab_menu1").addClass("port_back");
$("#tab_menu2, #tab_menu3").removeClass("port_back");
});
$("#tab_menu2").click(function(){
$(".tab_sub2").show();
$(".tab_sub1, .tab_sub3").hide();
$("#tab_menu2").addClass("port_back");
$("#tab_menu1, #tab_menu3").removeClass("port_back");
});
$("#tab_menu3").click(function(){
$(".tab_sub3").show();
$(".tab_sub1, .tab_sub2").hide();
$("#tab_menu3").addClass("port_back");
$("#tab_menu1, #tab_menu2").removeClass("port_back");
});
});
</script>
</head>
<body>
<div id="notice">
<ul>
<li id="tab_menu1" class="port_back">공지사항1</li>
<li id="tab_menu2">공지사항2</li>
<li id="tab_menu3">공지사항3</li>
</ul>
<div class="tab_list">
<div class="tab_sub1">서브1번입니다.<br>서브1번이라고요</div>
<div class="tab_sub2">서브2번입니다.<br>서브2번이라고요</div>
<div class="tab_sub3">서브3번입니다.<br>서브3번이라고요</div>
</div>
</div>
</body>
</html>
popup - javascript로 작성
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>popup - javascript</title>
<style>
html, body{
width: 100%;
height: 100%;
background-color: gold;
}
*{
font-family: "맑은 고딕";
}
#box{
width: 200px;
height: 200px;
background-color: red;
position: absolute;
top: 50%;
left: 50%;
display: none;
/* transform:translate(-50%, -50%);와 같음 */
margin-top: -100px;
margin-left: -100px;
}
</style>
</head>
<body>
<button id="a">Click</button>
<div id="box">
<h3>popup</h3>
<button id="cbtn">close</button>
</div>
<script>
document.getElementById('a').onclick=function(){
document.getElementById("box").style.display="block";
}
document.getElementById("cbtn").onclick=function(){
document.getElementById("box").style.display="none";
}
</script>
</body>
</html>
popup - jQuery로 작성
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="https://ajax.aspnetcdn.com/ajax/jQuery/jquery-3.6.0.min.js"></script>
<style>
html, body{
width: 100%;
height: 100%;
background-color: gold;
}
*{
font-family: "맑은 고딕";
}
#box{
width: 200px;
height: 200px;
position: absolute;
background-color: red;
top: 50%;
left: 50%;
display: none;
/* margin-top:-100px; margin-left:-100px; 이랑 같음 */
transform:translate(-50%, -50%);
}
</style>
</head>
<body>
<button id="a">Click</button>
<div id="box">
<h3>popup</h3>
<button id="cbtn">close</button>
</div>
<script>
$(document).ready(function(){
$("#a").click(function(){
$("#box").css("display","block");
});
$("#cbtn").click(function(){
$("#box").css("display","none");
});
});
</script>
</body>
</html>
popup이 열릴 때 뒤에 배경 어둡게
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="https://ajax.aspnetcdn.com/ajax/jQuery/jquery-3.6.0.min.js"></script>
<style>
html, body{
width: 100%;
height: 100%;
background-color: gold;
}
*{
font-family: "맑은 고딕";
}
#overlay_box{
width: 100vw;
height: 100vh;
background-color: rgba(0, 0, 0, 0.5);
position: fixed;
top: 0;
left: 0;
display: none;
}
#box{
width: 200px;
height: 200px;
position: absolute;
background-color: red;
top: 50%;
left: 50%;
/* margin-top:-100px; margin-left:-100px; 이랑 같음 */
transform:translate(-50%, -50%);
}
</style>
</head>
<body>
<button id="a">Click</button>
<div id="overlay_box">
<div id="box">
<h3>popup</h3>
<button id="cbtn">close</button>
</div>
</div>
<script>
$(document).ready(function(){
$("#a").click(function(){
$("#overlay_box").css("display","block");
});
$("#cbtn").click(function(){
$("#overlay_box").css("display","none");
});
});
</script>
</body>
</html>
☆ 모달과 팝업의 비교
- 모달
새로운 윈도우 창이 열리는 것이 아니라 레이어가 보여지는 것 (부모-자식 관계)
중간중간에 사용자에게 보여주는 경우가 많음.
display:none display:block
- 팝업
현재 열려있는 브라우저 페이지에 또 다른 브라우저 페이지를 위에 띄움
웹 사이트 시작과 동시에 띄우는 경우가 많음.
팝업창의 경우에는 사용자가 원할 경우 브라우저의 옵션을 통해 열지 않을 수 있음. 반드시 노출해야 하는 부분은 모달창을 사용하는 것이 좋음
cf. 사용자 관점
팝업창 - 현재 의도하는 목적과 상관없이 뜨는 창
모달창 - 다음 진행으로 넘어가기 위한 필요에 의해 사용되는 창
☆ top: 50%; left: 50%;
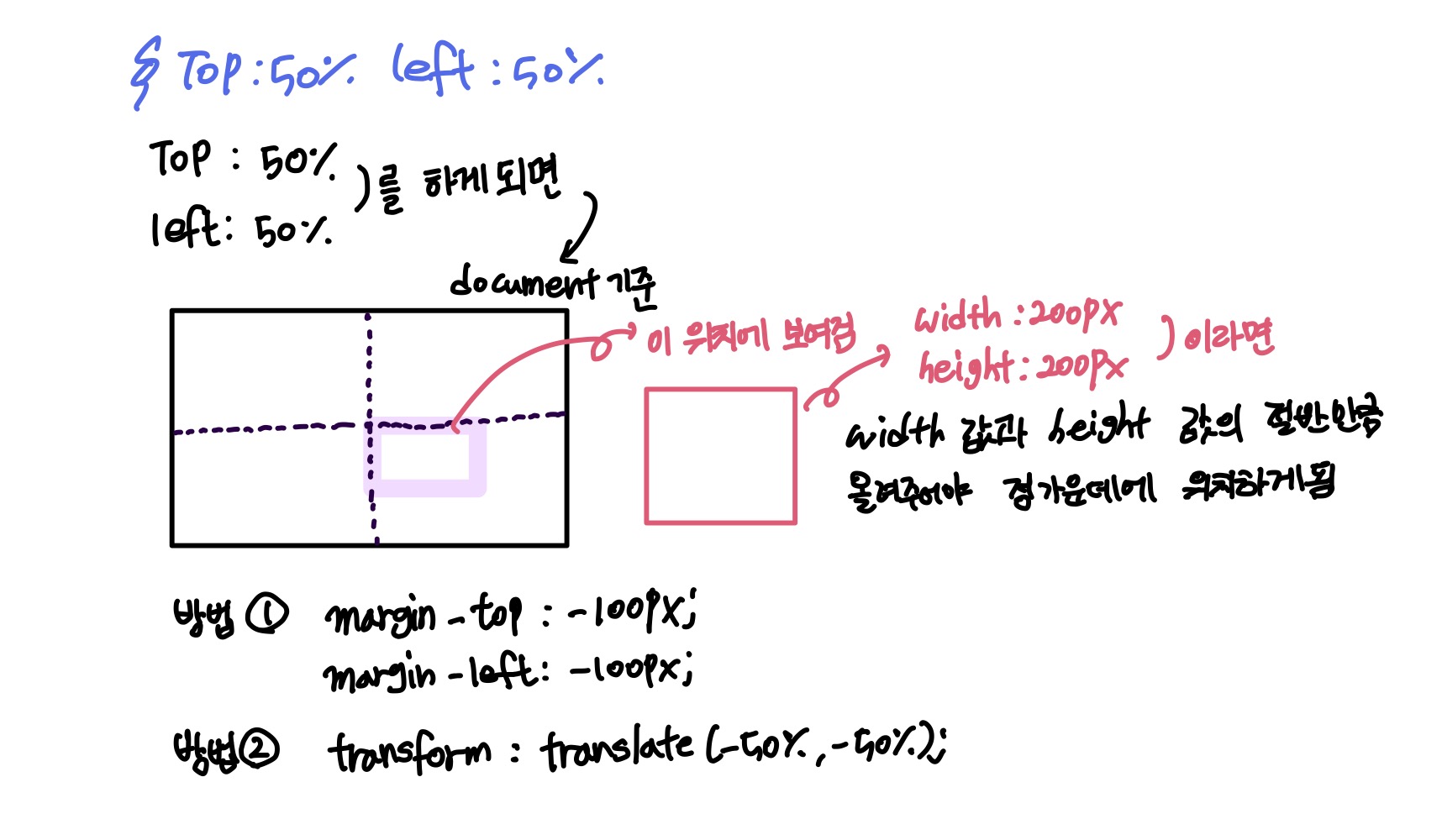
'자격증 > 웹디자인기능사' 카테고리의 다른 글
slide (0) | 2022.06.21 |
---|---|
웹디자인기능사 실기 - menu 모음 (0) | 2022.06.17 |
댓글